WooCommerce comes in with a whole lot of features and options but there are times when we need to customized a little further, just for example if we need to add an option to have our custom Meta Title and Meta Description or even an icon class or icon image URL. Now the question arises how to achieve this.
WordPress provides us with the 4 action which comes handy in this.
{taxonomy_name}_add_form_fields
=> to add fields in taxonomy create form{taxonomy_name}_edit_form_fields
=> to add fields in taxonomy edit formedited_{taxonomy_name}
=> to process edit form fieldscreate_{taxonomy_name}
=> to process create form fieldsmanage_edit-{taxonomy_name}_columns
=> to register custom column for admin screenmanage_{taxonomy_name}_custom_column
=> to populate content of custom column in admin screen
What is {taxonomy_name}
?
In WordPress, a taxonomy is a means of organizing (or categorizing) something. WordPress posts are configured by default with two taxonomies: "categories" and "tags". The values you create within those categories are known as its "terms". So {taxonomy_name}
stands for the slug that is associated with the taxonomy, for example: Post Categories => category
, Post Tag => post_tag
, Woo Product Categories => product_cat
, Woo Product Tags => product_tag
, etc..
Now let us get's our hands dirty.
<?php
add_action('product_cat_add_form_fields', 'wh_taxonomy_add_new_meta_field', 10, 1);
add_action('product_cat_edit_form_fields', 'wh_taxonomy_edit_meta_field', 10, 1);
//Product Cat Create page
function wh_taxonomy_add_new_meta_field() {
?>
<div class="form-field">
<label for="wh_meta_title"><?php _e('Meta Title', 'wh'); ?></label>
<input type="text" name="wh_meta_title" id="wh_meta_title">
<p class="description"><?php _e('Enter a meta title, <= 60 character', 'wh'); ?></p>
</div>
<div class="form-field">
<label for="wh_meta_desc"><?php _e('Meta Description', 'wh'); ?></label>
<textarea name="wh_meta_desc" id="wh_meta_desc"></textarea>
<p class="description"><?php _e('Enter a meta description, <= 160 character', 'wh'); ?></p>
</div>
<?php
}
//Product Cat Edit page
function wh_taxonomy_edit_meta_field($term) {
//getting term ID
$term_id = $term->term_id;
// retrieve the existing value(s) for this meta field.
$wh_meta_title = get_term_meta($term_id, 'wh_meta_title', true);
$wh_meta_desc = get_term_meta($term_id, 'wh_meta_desc', true);
?>
<tr class="form-field">
<th scope="row" valign="top"><label for="wh_meta_title"><?php _e('Meta Title', 'wh'); ?></label></th>
<td>
<input type="text" name="wh_meta_title" id="wh_meta_title" value="<?php echo esc_attr($wh_meta_title) ? esc_attr($wh_meta_title) : "; ?>">
<p class="description"><?php _e('Enter a meta title, <= 60 character', 'wh'); ?></p>
</td>
</tr>
<tr class="form-field">
<th scope="row" valign="top"><label for="wh_meta_desc"><?php _e('Meta Description', 'wh'); ?></label></th>
<td>
<textarea name="wh_meta_desc" id="wh_meta_desc"><?php echo esc_attr($wh_meta_desc) ? esc_attr($wh_meta_desc) : "; ?></textarea>
<p class="description"><?php _e('Enter a meta description', 'wh'); ?></p>
</td>
</tr>
<?php
}
Now that we have added form we need to handle the form request.
<?php
add_action('edited_product_cat', 'wh_save_taxonomy_custom_meta', 10, 1);
add_action('create_product_cat', 'wh_save_taxonomy_custom_meta', 10, 1);
// Save extra taxonomy fields callback function.
function wh_save_taxonomy_custom_meta($term_id) {
$wh_meta_title = filter_input(INPUT_POST, 'wh_meta_title');
$wh_meta_desc = filter_input(INPUT_POST, 'wh_meta_desc');
update_term_meta($term_id, 'wh_meta_title', $wh_meta_title);
update_term_meta($term_id, 'wh_meta_desc', $wh_meta_desc);
}
All the above code can be added into the active theme or child theme functions.php
file or also in any of our active plugin PHP files.
The above code will produce an output like this on the edit page.
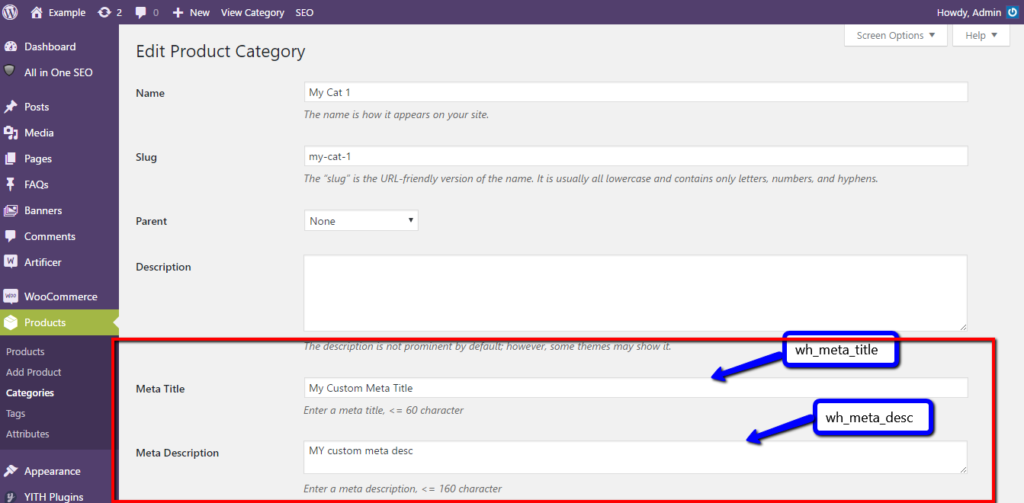
How to retrieve custom fields values which we have just created ?
<?php
$productCatMetaTitle = get_term_meta($term_id, 'wh_meta_title', true);
$productCatMetaDesc = get_term_meta($term_id, 'wh_meta_desc', true);
Now that we have learned as how to add this fields in Product Category, so if we what to add this in Post Category fields then we just have to replace product_cat
with category
from all the four mentioned action so action will look like some this like this. product_cat_add_form_fields
=> category_add_form_fields
and so on.
How to show custom fields values in Product Category Admin Screen listing?
<?php
//Displaying Additional Columns
add_filter( 'manage_edit-product_cat_columns', 'wh_customFieldsListTitle' ); //Register Function
add_action( 'manage_product_cat_custom_column', 'wh_customFieldsListDisplay' , 10, 3); //Populating the Columns
/**
* Meta Title and Description column added to category admin screen.
*
* @param mixed $columns
* @return array
*/
function wh_customFieldsListTitle( $columns ) {
$columns['pro_meta_title'] = __( 'Meta Title', 'woocommerce' );
$columns['pro_meta_desc'] = __( 'Meta Description', 'woocommerce' );
return $columns;
}
/**
* Meta Title and Description column value added to product category admin screen.
*
* @param string $columns
* @param string $column
* @param int $id term ID
*
* @return string
*/
function wh_customFieldsListDisplay( $columns, $column, $id ) {
if ( 'pro_meta_title' == $column ) {
$columns = esc_html( get_term_meta($id, 'wh_meta_title', true) );
}
elseif ( 'pro_meta_desc' == $column ) {
$columns = esc_html( get_term_meta($id, 'wh_meta_desc', true) );
}
return $columns;
}
Added functions in file functions.php by template, but where add code:
$productCatMetaTitle = get_term_meta($term_id, ‘wh_meta_title’, true);
$productCatMetaDesc = get_term_meta($term_id, ‘wh_meta_desc’, true);
?
Thank..
You have to
echo $productCatMetaTitle
where you want to display the data.it’s not working while i’m trying to show the $productCatMetaTitle where i want to show.. what is the problem? can you help me please?
Hello,
i am trying to send data. But forms are empty after saving edits.
Why? Thank you.
Hi Josef,
have you added
wh_save_taxonomy_custom_meta()
with create and editaction
?Highly energetic article, I liked that a lot.
Will there be a part 2?
Hi,
No there will not be any part 2 because it does not need that, but yes I’ll keep the article updated.
Normally I don’t learn post on blogs, however I wish to say that this write-up very forced me to try
and do it! Your writing style has been surprised me.
Thank you, quite nice post.
Hi Raunak,
I am trying to use your code for adding a few radio buttons. I want to use different templates for different different category pages.
I am able to add the radio buttons to the Cat Create page and Cat Edit page using the following (your code updated) :
//Product Cat Create page
function wh_taxonomy_add_new_meta_field() {
?>
Standard
Sprängskissmall
term_id;
// retrieve the existing value(s) for this meta field.
$wh_meta_category = get_term_meta($term_id, ‘wh_meta_category’, true);
// $wh_meta_desc = get_term_meta($term_id, ‘wh_meta_desc’, true);
?>
Standard
Sprängskissmall
<?php
}
add_action('product_cat_add_form_fields', 'wh_taxonomy_add_new_meta_field', 10, 1);
add_action('product_cat_edit_form_fields', 'wh_taxonomy_edit_meta_field', 10, 1);
// Save extra taxonomy fields callback function.
function wh_save_taxonomy_custom_meta($term_id) {
$wh_meta_category = filter_input(INPUT_POST, 'wh_meta_category');
update_term_meta($term_id, 'wh_meta_category', $wh_meta_category);
}
add_action('edited_product_cat', 'wh_save_taxonomy_custom_meta', 10, 1);
add_action('create_product_cat', 'wh_save_taxonomy_custom_meta', 10, 1);
However, the value is not saved when I check it.
Hi Peter,
Is your radio filed name is set to
name='wh_meta_category'
and what you get inwh_save_taxonomy_custom_meta()
method if you print the variableprint_r($wh_meta_category);
?Great, thanks!
But the only little thing helped me to retrieve the
meta_title
on the front isget_queried_object_id()
instead of your$term_id
:echo get_term_meta(get_queried_object_id(), 'wh_meta_title', true);
instead of
echo get_term_meta($term_id, 'wh_meta_title', true);
I added all your code, expecting (How to show custom fields values in Product Category Admin Screen listing?) part to functions.php.
How can I add displaying code into functions.php like HOOK?
Thank you.
Sorry didn’t get you on this. Do you want to know how to show the get the value and show it in frontend?
If so then you can use it like this
You can use this
$productCatMetaTitle = get_term_meta($term_id, 'wh_meta_title', true);
to retrive the value now you can display it were ever you want.Hi Raunak, I retrieved the value but how can I display it on frontend?
Hi @Michal,
After retriving you simply have to
echo
it.Yes but where is supose to be ?? Thanks?
Hi, I tried use this resolve, but when i use
echo $productCatMetaDesc
inarchive-product.php
i didnt get variable value. All code i added tofunction.php
file.Very Nice Tutorial! Working Perfectly Thanks!
One thing, As I have made checkbox custom field and it’s working fine on both create and edit screens. But when I create a new category with the checkbox checked then it’s not clear the checkbox value means it is not showing on the initial state with unchecked. Can you help with that?
Thanks Again!
Seriously guys… Could somebody correct the whole code?
I succeeded creating the meta fields and saving the content but I’m failing big time trying to actually hook these under the categories acordingly.
add_action( 'woocommerce_after_subcategory_title', 'blablabla', 5 );
function blablabla() {
echo get_term_meta(get_queried_object_id(), 'wh_meta_title', true);
}
Hi Nowhereman,
You want to show the value of custom field in sub-category as well?
Thanks for the tutorial , but I am getting this error :
Warning: call_user_func_array() expects parameter 1 to be a valid callback, function 'wh_taxonomy_edit_meta_field' not found or invalid
Hi Ahmed,
Have you added
add_action(‘product_cat_edit_form_fields’, ‘wh_taxonomy_edit_meta_field’, 10, 1);
in yourfunctions.php
fileHello, how can I return this amount of wh_meta_title in the api?
That is, in the wp-json/wc/v3/products/categories path
how can I get wh_meta_title
Hi Soheil,
You can add it by using
rest_api_init
action andregister_rest_field
filter and first param will be “product_cat”. Let me know if you still have any query then will try to give a full example.Oh wait – I forgot to activate the new plugin. You can delete that comment I made still in moderation and I’ll post a glowing one.
It DOES work! YAY!
Do you offer custom work? If so, please let me know how I can contact you via email to discuss.
Hi Mark,
You can reachout to us, via facebook or twitter.